PndMCTrackAnaExampleΒΆ
An example how to generate a requirements macro for the PndMCTrackAna Task
The following decay tree is taken as an example. It contains both charged and neutral final states as well as intermediate stages.
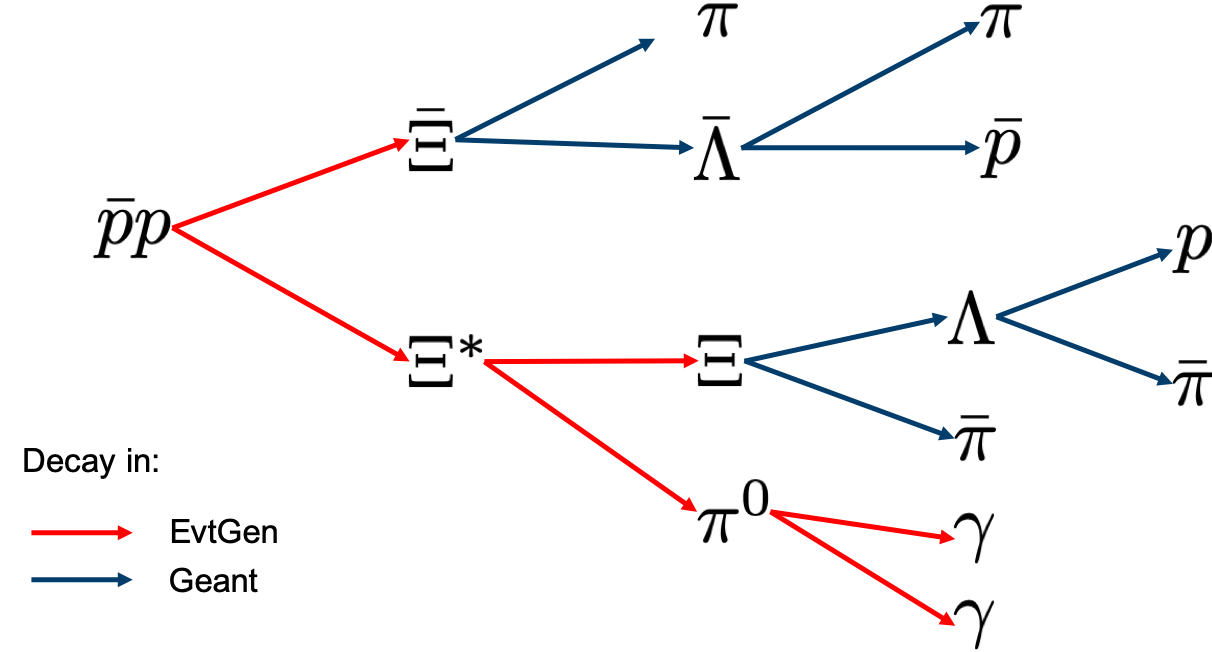
And here is the macro for the PndMCTrackInfoAna task:
1 int MCTrackInfoAna(Int_t nEvents = 0, TString prefix = "../data/evtcomplete")
2 {
3
4 // Number of events to process
5 // Int_t nEvents = 0; // if 0 all the vents will be processed
6
7 TString parAsciiFile = "all.par";
8 TString input = "psi2s_Jpsi2pi_Jpsi_mumu.dec";
9 TString output = "mctrackinfoana";
10 TString friend1 = "sim";
11 TString friend2 = "mctrackinfo";
12 TString friend3 = "";
13 TString friend4 = "";
14
15 // ----- Initial Settings --------------------------------------------
16 PndMasterRunAna *fRun = new PndMasterRunAna();
17 fRun->SetInput(input);
18 fRun->SetOutput(output);
19 fRun->AddFriend(friend1);
20 fRun->AddFriend(friend2);
21 fRun->AddFriend(friend3);
22 fRun->AddFriend(friend4);
23 fRun->SetParamAsciiFile(parAsciiFile);
24 fRun->Setup(prefix);
25
26 fRun->UseFairLinks(kTRUE);
27
28 FairGeane *Geane = new FairGeane();
29 fRun->AddTask(Geane);
30
31 // ------------------------------------------------------------------------
32
33 PndEventRequirements eventReq;
34
35 std::vector<int> mothers;
36 std::vector<int> daughters;
37
38 PndParticleRequirements LambdaBar;
39 LambdaBar.SetPID(-3122);
40 mothers = {-3312};
41 daughters = {-2212, 211};
42 LambdaBar.SetPIDMothers(mothers);
43 LambdaBar.SetPIDDaughters(daughters);
44 eventReq.AddTrackRequirement(LambdaBar);
45
46 PndParticleRequirements pBar;
47 pBar.SetPID(-2212);
48 mothers = {-3312, -3122};
49 pBar.SetPIDMothers(mothers);
50 pBar.SetCheckTracking("Barrel", "FtsIdeal", "Proton");
51 eventReq.AddTrackRequirement(pBar);
52
53 PndParticleRequirements pi1;
54 pi1.SetPID(211);
55 mothers = {-3312, -3122};
56 pi1.SetPIDMothers(mothers);
57 pi1.SetCheckTracking("Barrel", "FtsIdeal", "Pion");
58 eventReq.AddTrackRequirement(pi1);
59
60 PndParticleRequirements pi2;
61 pi2.SetPID(211);
62 mothers = {-3312};
63 pi2.SetPIDMothers(mothers);
64 pi2.SetCheckTracking("Barrel", "FtsIdeal", "Pion");
65 eventReq.AddTrackRequirement(pi2);
66
67 PndParticleRequirements Lambda;
68 Lambda.SetPID(3122);
69 mothers = {3312};
70 daughters = {2212, -211};
71 Lambda.SetPIDMothers(mothers);
72 Lambda.SetPIDDaughters(daughters);
73 eventReq.AddTrackRequirement(Lambda);
74
75 PndParticleRequirements p;
76 p.SetPID(2212);
77 mothers = {3312, 3122};
78 p.SetPIDMothers(mothers);
79 p.SetCheckTracking("Barrel", "FtsIdeal", "Proton");
80 // p.SetCheckPid("PidAlgoMvdProton;PidAlgoSttProton;PidAlgoDrcProton;PidAlgoDiscProton;PidAlgoEmcBayesProton;PidAlgoSciTProton;PidAlgoFtofProton", 0.99);
81 eventReq.AddTrackRequirement(p);
82
83 PndParticleRequirements piBar1;
84 piBar1.SetPID(-211);
85 mothers = {3312, 3122};
86 piBar1.SetPIDMothers(mothers);
87 piBar1.SetCheckTracking("Barrel", "FtsIdeal", "Pion");
88 eventReq.AddTrackRequirement(piBar1);
89
90 PndParticleRequirements piBar2;
91 piBar2.SetPID(-211);
92 mothers = {3312};
93 piBar2.SetPIDMothers(mothers);
94 piBar2.SetCheckTracking("Barrel", "FtsIdeal", "Pion");
95 eventReq.AddTrackRequirement(piBar2);
96
97 PndParticleRequirements gamma1;
98 gamma1.SetPID(22);
99 gamma1.SetCheckNeutral();
100 eventReq.AddTrackRequirement(gamma1);
101
102 eventReq.AddTrackRequirement(gamma1); // two photons from the pi0 decay
103
104 PndMCTrackInfoAnaTask *trackInfo = new PndMCTrackInfoAnaTask();
105 trackInfo->SetEventRequirements(eventReq);
106 fRun->AddTask(trackInfo);
107
108 // ----- Intialise and run --------------------------------------------
109
110 cout << "fRun->Init()" << endl;
111
112 fRun->Init();
113 fRun->Run(0, nEvents);
114 // ------------------------------------------------------------------------
115
116 return 0;
117 }